Simple Model Example
This example explain how to apply X-O lite XML serialization/deserialization to a simple java object model.
This example models a company. It's just a data-oriented model where you can store a Company, it's address the list of employees and contractors working in the company and their private address. It's a very simplified model but it already demonstrates two object behaviors:
- Composition & reuse: The model is a tree of interrelated objects, in particular the Address object is used a two different places
- Inheritance: Both the Employee and the Contractor objects inherit from the Person object.
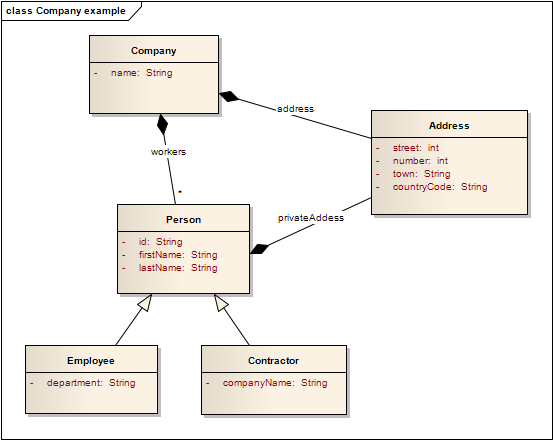
This model is mapped to a XML like:
<?xml version="1.0"?>
<company xmlns="xo-lite.sf.net/examples/company">
<name>Test Company 2</name>
<address>
<street>Grand rue</street>
<number>123</number>
<town>Brussels</town>
<country>Belgium</country>
</address>
<workers>
<employee id="E1">
<name>
<firstName>Bill</firstName>
<lastName>Foo</lastName>
</name>
<privateAddress>
<street>Plattestraat</street>
<number>45</number>
<town>Zotteghem</town>
<country>Belgium</country>
</privateAddress>
<department>ICT</department>
</employee>
<contractor id="C1">
<name>
<firstName>Joe</firstName>
<lastName>Bar</lastName>
</name>
<privateAddress>
<street>Dr�ve du bois</street>
<number>6</number>
<town>Waterloo</town>
<country>Belgium</country>
</privateAddress>
<companyName>ICT pro S.A.</companyName>
</contractor>
(... other employees or contractors ...)
</workers>
</company>
This is a simple XML but it already have some particularities that can make it difficult to bind to java objects:
- The same <name> tag appear at two places with two different maening and structures.
- Some XML elements are not represented in the Java objects (like <workers> or <name> inside employee). They just exist in the XML to bring more structure/clarity.
- The same java objects (the Address) is mapped at two different places using two different XML tags (<address> and <privateAddress>
Source files
The complete source code of this example is located in the:
xo-lite-1.0/src-projects/xo-lite-examples/src/main/java/net/sf/xolite/company
directory inside the xo-lite-1.0-all.zip or xo-lite-1.0-all.tgz distribution archive.
To build and run all the examples:
- Extract the distribution archive in any directory.
- ensure you have Maven latest version installed and configured on your computer.
- issue the "mvn compile exec:java" shell command from the .../xo-lite-1.0/src-projects/xo-lite-examples directory.
Implementation Description
Mapping this model to XML is a very regular case for X-O lite. There is nothing special in the model objects XMLSerializable implementation
You can just note the boolean passed to the Address object in it's constructor so that it can know if it is used as a company address or as the private address of a person (and hence choose the correct tag when serialized).
For this implementation, as an example, we've made the choice to validate the input XML in the parsing code (although it is also checked by the internal parser via the XML schema). This way you can turn of the parser schema validation and still have a 'minimum' validation. But, it requires some extra code.
Results
Though this simple project is not the best target for X-O lite applicability (because objects are merely data holders) you can already see the benefits of X-O lite Object Oriented approach.
- Strictly private data: in the whole model all object data are private without any getter and setter. Even in the inheritance pattern, the data of the ancestor class are private without accessor and still play well in XML parsing/serializing.
Note: this doesn't mean that you cannot write getters/setters if you need them for other purpose, it just means that X-O lite strictly respect encapsulation. - Private inner XML constants: most of the string constants used for XML tags and attribute names can remain private. The only that have to be public is the outer most XML tag of the object (which is somehow part of it's interface). The other constants like attribute names can remain private. It means that you can easily modify an object and the way it is represented in XML without affecting the others.
- Inheritance: The object inheritance scheme fits well with X-O lite, the parsing/serialization of a parent class is written in the class and inherited in sub-classes. In this example, you can see that the code parsing/serializing the Person data is not duplicated in each sub-class.
Note: we will see in the recursive model example a more complex inheritance scheme where polymorphism is used in both objects and XML schema.
- Composition & Reuse: As they don't see their parent and there is no dependency on the XML context, the objects are easy to compose. In particular, the same object (here the address) can be reused in several context inside the XML.
- Locality: The XML tags have other meanings and syntax depending on their position in the XML (like the <name> tag in the example) do not cause problem. It's because each object parses its own data which is locally not ambiguous.
This example also demonstrates that X-O lite plays well with java XML APIs.
- Enabling schema validation is easy
- Support of XML namespaces is easy (as you don't have to bother with prefix mappings).